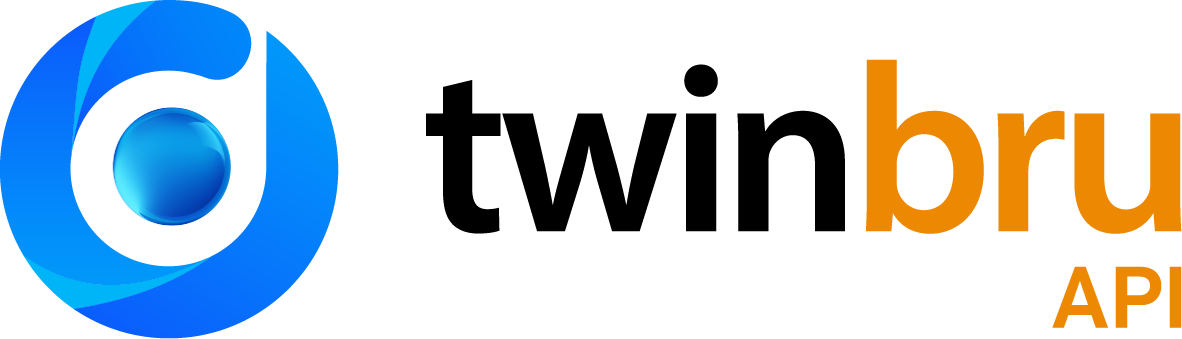
Example
HTTP
GET https://api.twinbru.com/products
Ocp-Apim-Subscription-Key:
{subscriptionKey}Authorization:
Bearer {token}
Api-Version: v1
Curl
curl -v GET "https://api.twinbru.com/products" -H "Api-Version: v1" -H "Ocp-Apim-Subscription-Key: your-subscription-key" -H "Authorization: Bearer your-bearer-token"
C#
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Threading.Tasks;
namespace ODSAPISample
{
class Program
{
const string subscriptionKey = "your-subscription-key";
const string token = "your-bearer-token";
static async Task Main(string[] args)
{
var client = new HttpClient();
var uri = "https://api.twinbru.com/products";
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token);
client.DefaultRequestHeaders.Add("Ocp-Apim-Subscription-Key", subscriptionKey);
client.DefaultRequestHeaders.Add("Api-Version", "v1");
var response = await client.GetAsync(uri);
var jsonResult = await response.Content.ReadAsStringAsync();
Console.WriteLine(jsonResult);
Console.ReadLine();
}
}
}
Java
import java.net.URI;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class JavaSample
{
public static void main(String[] args)
{
HttpClient httpclient = HttpClients.createDefault();
try
{
URIBuilder builder = new URIBuilder("https://api.twinbru.com/products");
URI uri = builder.build();
HttpGet request = new HttpGet(uri);
request.setHeader("Cache-Control", "no-cache");
request.setHeader("Api-Version", "v1");
request.setHeader("Ocp-Apim-Subscription-Key", "your-subscription-key");
request.setHeader("Authorization", "Bearer your-brearer-token");
HttpResponse response = httpclient.execute(request);
HttpEntity entity = response.getEntity();
if (entity != null)
{
System.out.println(EntityUtils.toString(entity));
}
}
catch (Exception e)
{
System.out.println(e.getMessage());
}
}
}
Python
import httplib, urllib, base64
headers = {
# Request headers
'Cache-Control': 'no-cache',
'Api-Version': 'v1',
'Ocp-Apim-Subscription-Key': 'your-subscription-key',
'Authorization': 'your-bearer-token',
}
params = urllib.urlencode({})
try:
conn.request("GET", "?%s" % params, headers)
response = conn.getresponse()
data = response.read()
print(data)
conn.close()
except Exception as e:
print("[Errno {0}] {1}".format(e.errno, e.strerror))
Authentication
The API authentication requires a bearer token and a subscription key.
For each request to the API, both headers must be provided as shown in the example.
Subscription key can be requested within one of the products. After selecting a product, ex: Bru Products, a subscribe button is available. Upon clicking, a subscription key will be displayed in your profile page. However, it will need to be manually activated by Bru.
When the subscription key is activated, a bearer token will be manually generated as well and sent by e-mail.
If the subscription key or token is invalid, a proper error message will be returned.
Aside from authentication headers, a third header, Api-Version is also required for each request to the API, with a value of v1.
Additionally to the following examples, there's a Postman collection also available at this link:
https://www.postman.com/collections/2c3ae85009c1031471de
You can see a postman collection by opening Postman, click Import and paste the previous url into the Link tab. Be sure to define your bearer token and subscription variables before running any requests in the collection.